The Maze
Question
Given a maze represented by a matrix, start position of a ball, and the goal position, determine if it's possible for the ball to stop at the goal position.
- Rules:
- A cell that has "1" is a wall
- A cell that has "0" is an empty space
- The ball cannot stop rolling until it hits a wall
- The ball cannot change directions until it hits a wall
- Any cells outside the boundaries of the matrix can assumed to be walls
Input: maze = [ [0, 0, 0, 0], [1, 0, 1, 0], [1, 0, 0, 0], [0, 0, 0, 0] ], start = (0, 0), goal = (3, 0)
Output: True
One of the paths the ball can move is (0, 0) -> (0, 3) -> (3, 3) -> (3, 0).
Input: maze = [ [0, 0, 0, 0], [1, 0, 1, 0], [1, 0, 0, 1], [0, 0, 0, 0], ], start = (0, 0), goal = (3, 0)
Output: False
The ball would not be able to roll from the start position to goal position.
Input: maze = [ [1, 0, 0, 1, 0], [0, 0, 0, 0, 0], [0, 0, 0, 1, 0], [0, 0, 0, 0, 0], [0, 1, 0, 0, 1] ], start = (0, 1), goal = (4, 0)
Output: True
One of the paths the ball can move is (0, 1) -> (3, 1) -> (3, 0) -> (4, 0).
Clarify the problem
What are some questions you'd ask an interviewer?
Understand the problem
maze = [
[0, 0, 1, 1]
[1, 0, 0, 0]
[0, 0, 0, 0]
[0, 0, 1, 0]
]
start = (0, 0)
end = (3, 3)
Login or signup to save your code.
Uh oh... looks like you don't yet have access.
Not sure what this unlocks? Check out a free pattern section.
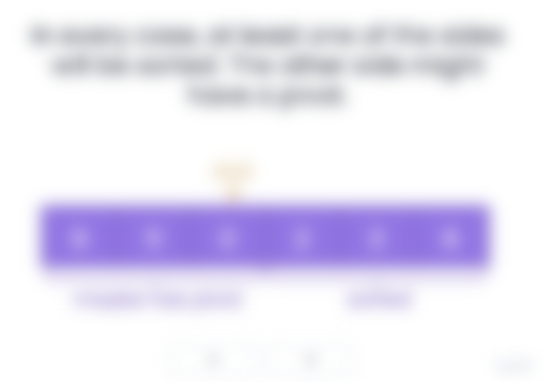
Example solution
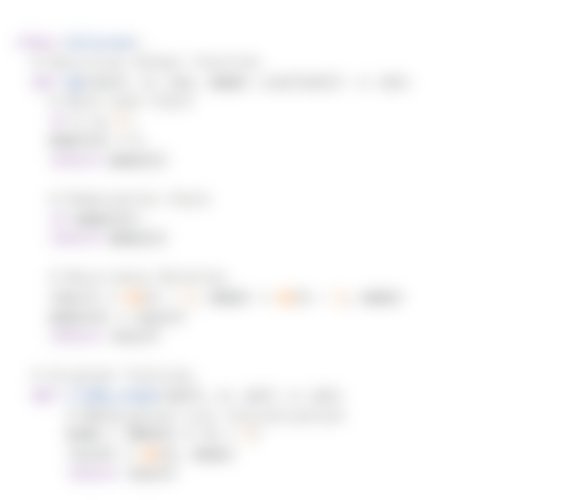
Complexity
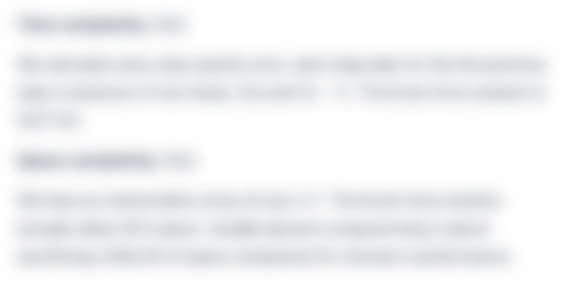